The Rule of Delete: A Simple Test for Code Organization
If removing a feature takes more than three delete actions, your code might be too tangled. In this article, Adnan introduces the Rule of Delete - a practical test for modularity and maintainability. Learn how this simple approach aligns with core software design principles.
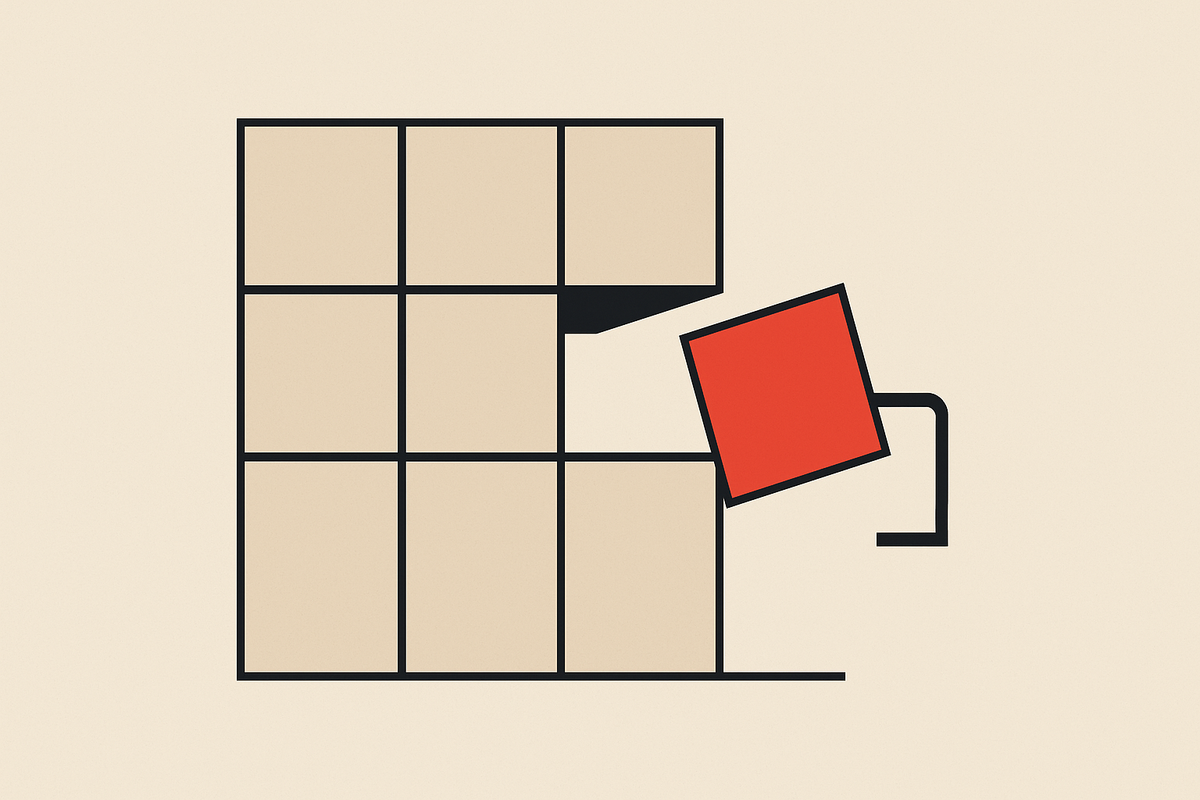
Motivation
Recently, I came across several articles discussing the trend of moving from a microservices architecture back to a monolithic one, often for a variety of practical reasons. In most of these cases, a modular monolith is presented as a viable alternative to microservices. Interestingly, some large companies have transitioned away from microservices in favor of a monolithic approach, while others have used a monolith from the beginning and never adopted microservices at all.
This resonates with my own belief that, in many cases, microservices are introduced not out of genuine necessity, but rather because some technical stakeholders (developers, engineers) feel compelled to demonstrate their ability to work with them — much like an overconfident drinker trying to prove they can outdrink everyone else.
That said, I’m not here to dive into architectural debates. Instead, I want to focus on a particular aspect of code organization — one that might remind you of a modular approach, even if it isn’t strictly architectural.
Introduction
Good code isn’t just about writing new features—it’s about making changes easy. Whether it’s adding a new feature, modifying existing functionality, or removing something entirely, a well-structured codebase should make these tasks simple. But how do you evaluate if your code is well-organized?
This article introduces the Rule of Delete, a simple heuristic for assessing code structure. The idea is straightforward:
After implementing a new feature or a module, imagine you need to remove all of its related code. If you can do this in three or fewer delete actions, your code is likely well-structured. If it takes significantly more, your code may be overly coupled, scattered, or difficult to maintain.
This rule provides a quick, intuitive way to assess code organization. The value of this rule lies in its simplicity and practicality. It’s not about enforcing strict deletion limits but about highlighting modularity, separation of concerns, and maintainability.
The idea behind the Rule of Delete is simple: a well-structured feature should be self-contained and easy to remove. If deleting a feature requires modifying multiple unrelated files, it suggests the code is too entangled with the rest of the system.
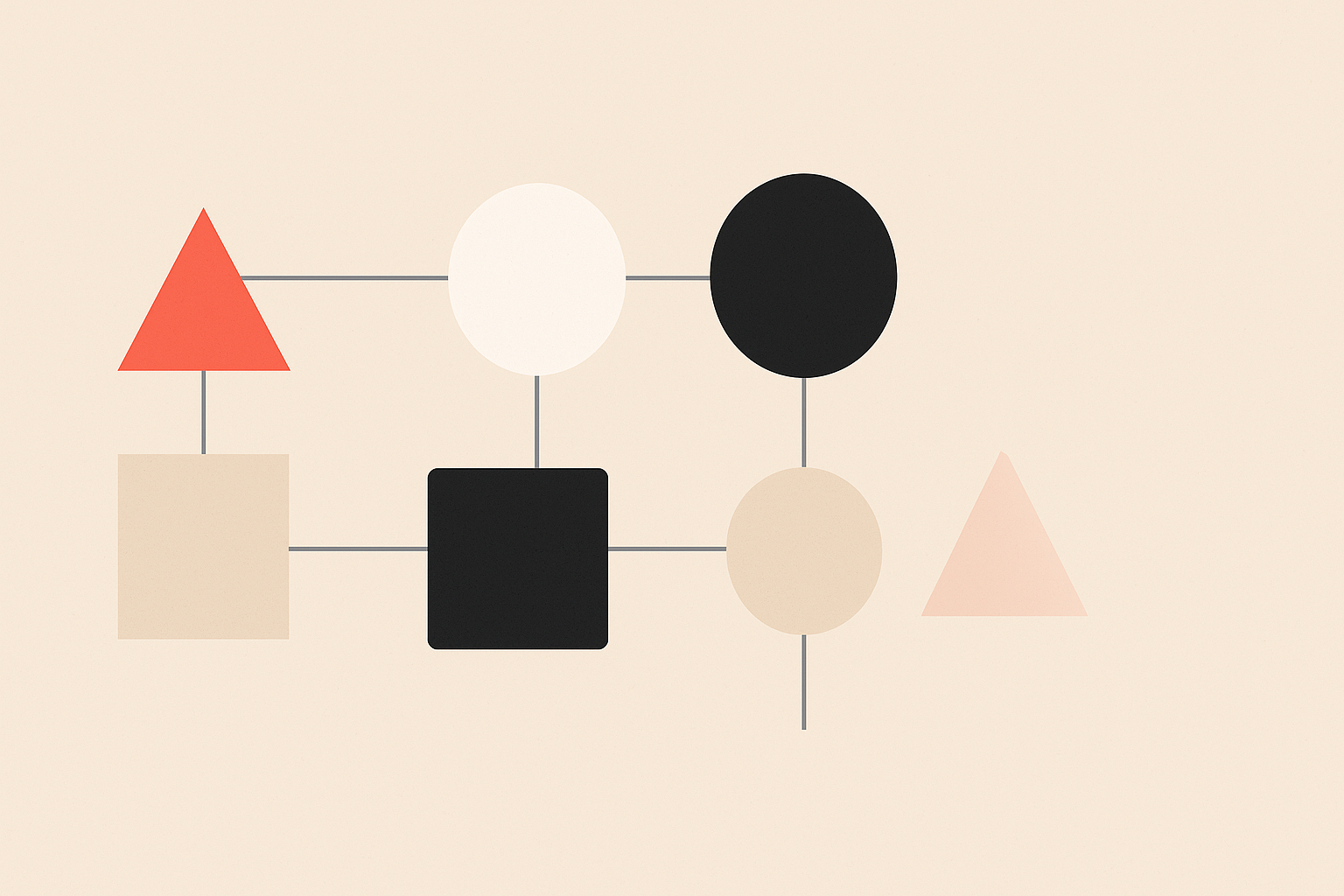
The Rule of Delete Explained
Here’s how to apply the Rule of Delete in practice:
- Implement a new feature as you normally would.
- Imagine that you need to remove it completely—pretend it was a bad idea or no longer needed.
- Count how many delete actions are required to fully remove all related code.
What Counts as a "Delete Action"?
A delete action is a single logical removal of a feature-related unit. For example:
✅ One delete action: Removing a dedicated module or directory containing all the feature’s code.
✅ One delete action: Removing a well-encapsulated class, component, or API endpoint.
✅ One delete action: Deleting a single database table or schema related to the feature.
🚫 Multiple delete actions required: If removal involves modifying unrelated files across multiple layers of the application, the structure needs improvement.
Nothing new
The Rule of Delete is not a new concept—it aligns with several well-known software design principles. The difference? It simplifies them into an easy-to-remember, practical test, especially useful for newcomers. Here are few well-known principles:
The Open-Closed Principle (OCP)
- Definition: Software entities should be open for extension but closed for modification.
- Connection to Rule of Delete: If removing a feature requires modifying multiple unrelated parts of the code, it likely violates OCP. A well-structured system should allow features to be removed cleanly, not require modifying core logic.
The Single Responsibility Principle (SRP)
- Definition: A class or module should have only one reason to change—it should encapsulate a single responsibility.
- Connection to Rule of Delete: If a feature’s logic is scattered across multiple components that serve different purposes, it’s a sign of poor structure. The Rule of Delete encourages modular feature design, indirectly reinforcing SRP.
More Principles to Consider
You can easily map the Rule of Delete to several other design principles as well — here are a few worth exploring:
- The Law of Demeter
- Plugin Architecture & Inversion of Control
- Feature Toggles & the Strangler Pattern
Final thoughts
While the Rule of Delete is a useful heuristic, it should not be taken as an absolute rule. Context always matters. Some features naturally require integration across multiple components, and forcing a strict three-delete limit might not always be practical.
The real value of this rule is not in rigid enforcement but in serving as an indicator—if removing a feature feels excessively complicated, it’s a sign that your code organization might need improvement.
Its strength lies in its practicality—it gives developers an immediate, tangible way to evaluate code organization. It helps highlight potential issues, but good engineering judgment is still required to determine whether restructuring is truly necessary.
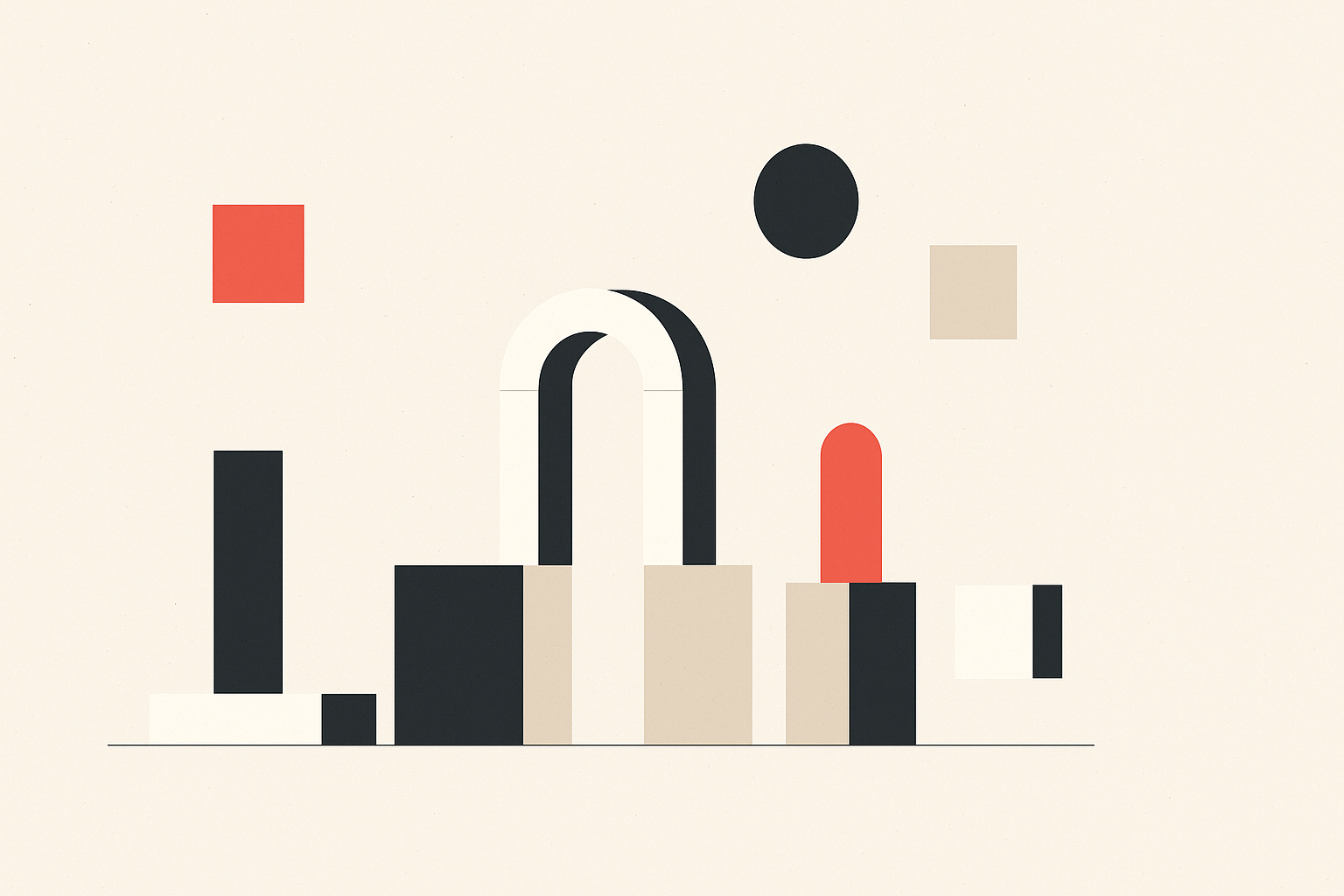
Next time you implement a new feature, ask yourself:
If I had to delete this, how hard would it be?
If the answer involves more than three delete actions, it might be time for a refactor.